Node.js で使用してCSVファイルを受け取り、その内容をパースしてデータベースのテーブルに登録する実装方法を解説します。
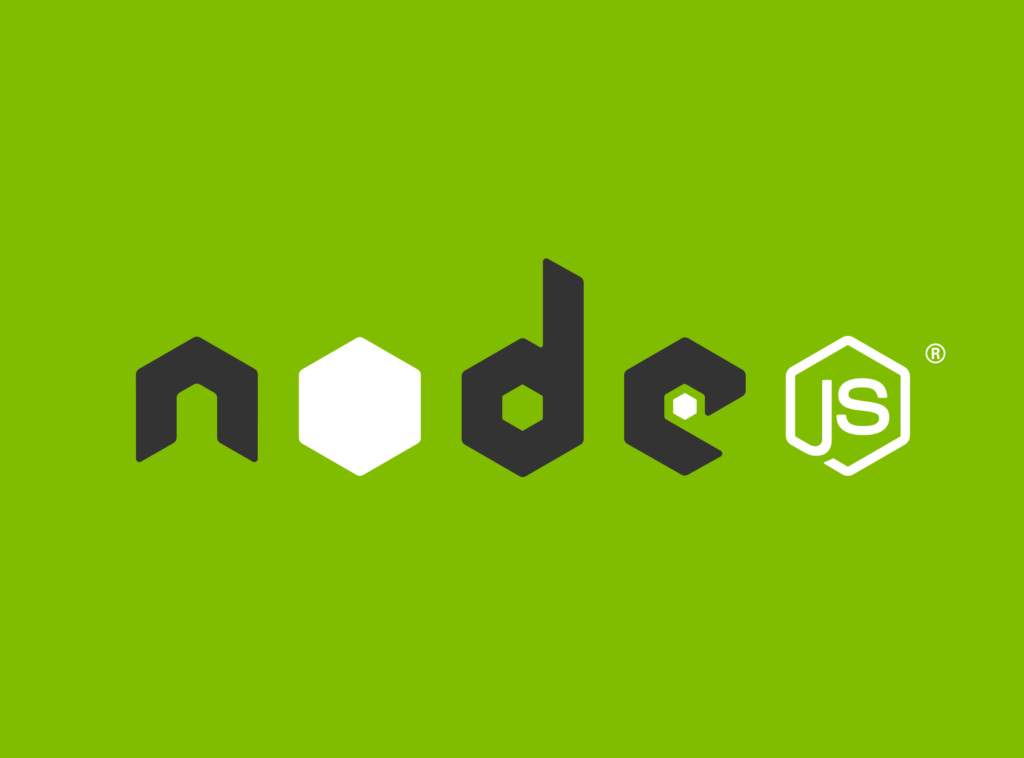
CSVファイルのパースとDBへの登録 実装方法
以下は、Node.jsとExpress.jsを使用してCSVファイルを受け取り、その内容をパースしてデータベースのテーブルに登録する実装例になります。
まず、必要なパッケージをインストールします。
npm install express pg csv-parser multer
次に、以下でCSVファイルのパースとDBへの登録を行うAPIエンドポイントを作成します。
const express = require('express');
const { Pool } = require('pg');
const csv = require('csv-parser');
const multer = require('multer');
const app = express();
const dbConfig = {
user: 'your_username',
password: 'your_password',
host: 'localhost',
port: 5432,
database: 'your_database'
};
// ファイルのアップロードにmulterを使用
const upload = multer();
// POSTリクエストを処理するエンドポイント
app.post('/upload', upload.single('file'), async (req, res) => {
try {
// CSVファイルのストリームを取得
const fileStream = req.file.buffer;
// データベース接続
const pool = new Pool(dbConfig);
const client = await pool.connect();
// CSVパース処理とデータベースへの登録
fileStream
.pipe(csv({ separator: ',' }))
.on('data', async (data) => {
// データベースにデータを挿入
const insertQuery = {
text: 'INSERT INTO your_table (column1, column2) VALUES ($1, $2)',
values: [data.column1, data.column2] // カラム名に適切な値を指定
};
await client.query(insertQuery);
})
.on('end', () => {
// パースが完了したら成功を返す
res.json({ success: true });
})
.on('error', (err) => {
// エラーハンドリング
console.error(err);
res.status(500).send('An error occurred');
})
.on('close', async () => {
// データベース接続を閉じる
await client.release();
await pool.end();
});
} catch (error) {
// エラーハンドリング
console.error(error);
res.status(500).send('An error occurred');
}
});
// サーバーを起動
app.listen(3000, () => {
console.log('Server is listening on port 3000');
});
上記コードでは、以下の手順でCSVファイルをパースしてデータベースに登録します。
/upload
エンドポイントで、upload.single('file')
ミドルウェアを使用してCSVファイルを受け取ります。- 受け取ったCSVファイルのストリームを取得します。
- データベースに接続します。
- CSVファイルのストリームをパースし、データを1行ずつ取得します。
- 取得したデータをデータベースのテーブルに登録します。INSERTクエリのカラム名と値は適宜変更してください。
- パースが完了したら、成功のレスポンスを返します。
- エラーハンドリングとデータベース接続の終了処理も行います。
データベースの接続情報やテーブル名、カラム名は、dbConfig
オブジェクトとINSERTクエリの部分を適切に変更する必要があります。
また、安全性やパフォーマンスの向上のために、適切なエラーハンドリングやバリデーション、トランザクションの使用を検討する必要もあるのでご注意ください。